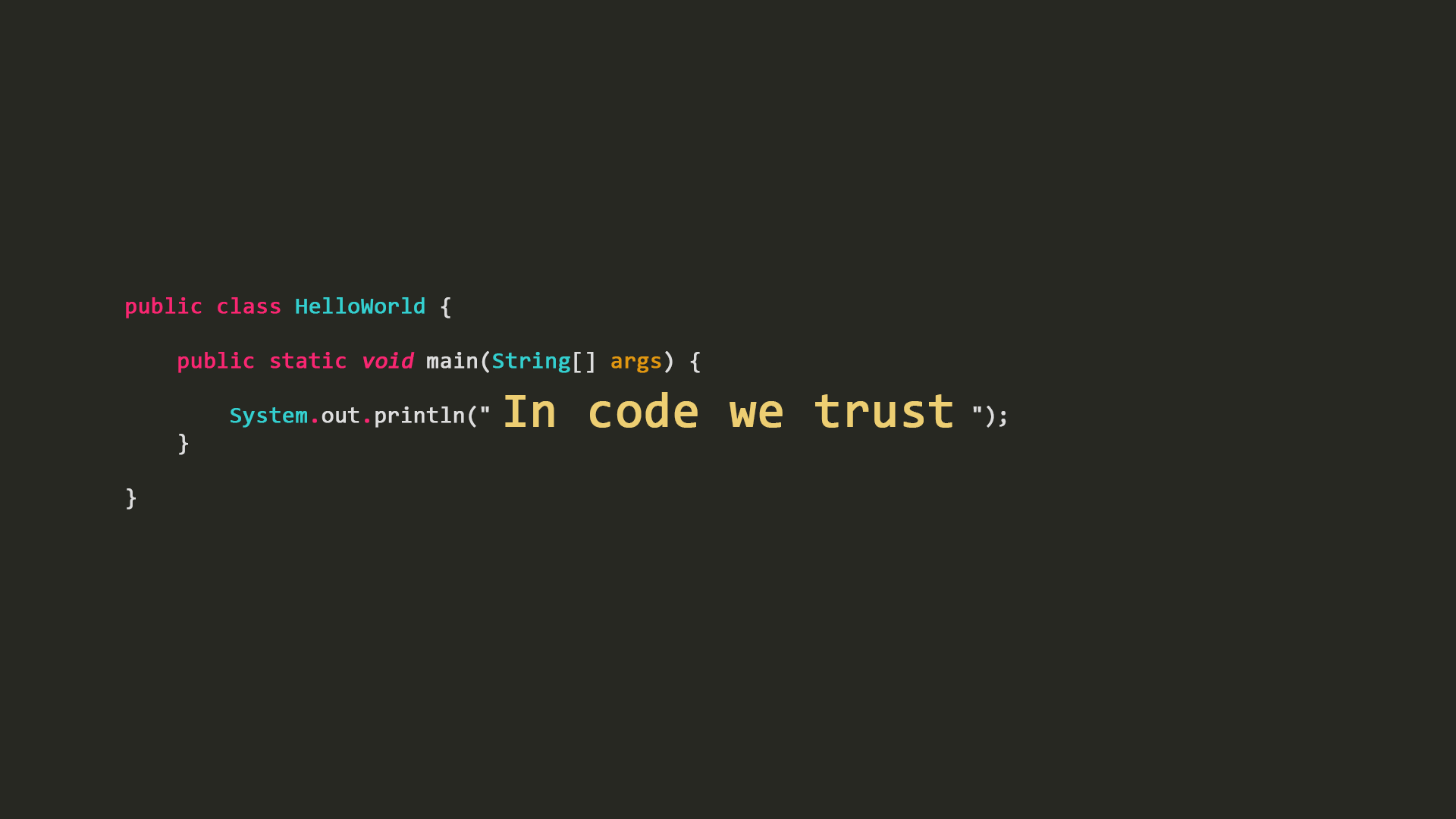
Reuse java classes
When programming, reuse a class, a module or a method is not rarely. If it simple and short, we can rewrite it, or copy it.
But it is long and complex, we couldn’t spend a lot of time to rewrite a class - which we have written before. We can copy the whole files from this project to another project, but it will be bulky and raise some issues how to put it in. That is, we can do it with jar
command, which command includes in bin
folder of your jdk
folder.
jar command
syntax to use:
jar [OPTION ...] [ [--release VERSION] [-C dir] files] ...
Example:
jar cvf your_library.jar UsefulThing.class
Output of this command will produce a jar file with name your_library.jar
And in the project, which you want to use this class, all thing you need todo is just add this jar file as a reference (detail how to do it, it will be different depend on your IDE, please search google).
And when run a java program with this jar file as a reference, we must include it in -classpath
option, view detail in: https://docs.oracle.com/javase/8/docs/technotes/tools/windows/classpath.html
packaging java class has package
To easy to manage the project, we usually (or almost) put every java class in a package. Example class:
package app.netlify.kuby_block.util;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateUtil {
private static SimpleDateFormat dateFormatter = new SimpleDateFormat();
public static Date parseDate(String formattedDate, String pattern) {
Date result = null;
try {
dateFormatter.applyPattern(pattern);
result = dateFormatter.parse(formattedDate);
} catch (ParseException e) {
e.printStackTrace();
}
return result;
}
public static String formatDate(Date inpDate, String pattern) {
String result = "";
dateFormatter.applyPattern(pattern);
if (null != inpDate) {
result = dateFormatter.format(inpDate);
}
return result;
}
}
In this example, we have DateUtil
class, and it exists in package app.netlify.kuby_block.util
.
When you jar this package, please enter to compiled folder of this module and do it. You should do it in another place, because it will create waste folder or lost folder, and raise unexpected error when reuse.
In my example, my structure folder will look like:
I will cd
to out/production/testingIntellij
, and run command:
jar cvf util.jar app
That all, it will create a file jar util.jar
with structure similar as I put in my project. And I can use it in any projects.
build executable jar file
We would like to use jar file as an executable file for many purposes, The way to do:
jar -cmf <path to mainifest file> <output jar file> <folder to package to executable jar file>
Example:
We go to the output folder: cd out/production/testingIntellij
jar -cmf META-INF/MAINIFEST.MF app.jar app/
And MAINIFEST.MF
file is
Manifest-Version: 1.0
Main-Class: app.netlify.kuby_block.Main
How to use it:
java -jar app.jar argument1 argument2
Example of using executable jar file with use another class in main class. Main.java will be used to parse date:
package app.netlify.kuby_block;
import app.netlify.kuby_block.util.DateUtil;
import java.util.Date;
public class Main {
public static void main(String[] args) {
if (0 < args.length) {
String rawDate = args[0];
final Date date = DateUtil.parseDate(rawDate, "yyyy-MM-dd");
System.out.println("parse date: " + date);
}
}
}
And use it after creating executable jar:
$ java -jar app.jar 2021-09-11
>> parse date: Sat Sep 11 00:00:00 ICT 2021
Actually, jar will do the same as compress files, except that we can create manifest file and manage version with it. But if you don’t care about version, we can use program zip file to do it, like “7zip”, “WinRar”, we compress folder or files we want and rename it with extension .jar
, it will be done for you. Carefully, you have to self manage folder and which folder be compressed to achieve what you want.
You should visit this page for detail: https://docs.oracle.com/en/java/javase/14/docs/specs/man/jar.html
Packaging with maven
If you familar with maven
, you can package your project with command mvn package
.
Additional infomation about your project and how to package it, you can config in pom.xml
file.
Useful? Yes, I think this feature is very cool and help developers to manage their project better.
You want to know more? Link here: https://maven.apache.org/guides/introduction/introduction-to-the-lifecycle.html