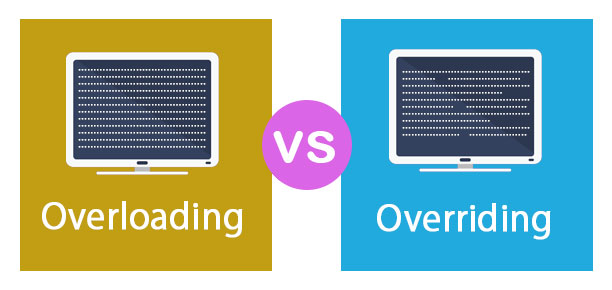
Overloading and overriding are two important concepts, not only in java language but in programming too.
Definitions
Overloading
is concept about the methods have the same method name but different parameters. It is not just in class (Object Oriented Programming - OOP), but in any programing language.
Overriding
is concept about the methods have the same method name and parameters as well. It only occurs in OOP, when a method of child class has the same method name and parameters with its parent class.
Overloading
You can create a overloading method in two ways:
- different about number of parameter
- different about type of parameter
Example:
public class MyClass {
public String method(){
return "method with no parameter";
}
public String method(String param1){
return "method with one parameter";
}
public String method(int param1){
return "method with an int parameter";
}
}
And we call it from another class:
public class App {
public static void main(String[] args) {
MyClass myClass = new MyClass();
String result1 = myClass.method();
String result2 = myClass.method("parameterVal");
String result3 = myClass.method(1);
System.out.println("result1: " + result1); // result1: method with no parameter
System.out.println("result2: " + result2); // result2: method with one parameter
System.out.println("result3: " + result3); // result3: method with an int parameter
}
}
Reference type will determine which overloaded method will be used at compile-time depends on what you give it.
Dive in
We have a tricky thing about overloading is, what method will be invoked in these examples:
public class App {
private static void doSomething(MyClass myClass){
System.out.println("invoked: myClass");
}
private static void doSomething(MyChildClass myClass){
System.out.println("invoked: myChildClass");
}
public static void main(String[] args) {
MyClass myClass = new MyChildClass();
doSomething(new MyClass()); // invoked: myClass
doSomething(new MyChildClass()); // invoked: myChildClass
doSomething(myClass); // invoked: myClass - it just cares about what you declare it and in this example, is `MyClass`
}
}
public class App {
private static void doSomething(MyClass myClass){
System.out.println("invoked: myClass");
}
private static void doSomething(MyChildClass myClass){
System.out.println("invoked: myChildClass");
}
public static void main(String[] args) {
MyClass myClass = new MyChildClass();
doSomething(myClass); // invoked: myClass
doSomething(null); // invoked: myChildClass
}
}
Because null
maybe a reference of either Object
, MyClass
or MyChildClass
, so java will choose what most specific class, in this case will be MyChildClass
.
Overriding
Overriding only exists in OOP programming, when you have a parent class and a child class which the same method name and paramters. Re-define method in child class is called override
, use can use annotation @Override
(in java) to clarify it.
Overriding is one of some features allow you to take advantage of polymorphism when designing your class. The overriden method of parent class will be hidden method by its child, and we only invoked it by super
keyword.
Example (continue with above example):
public class MyChildClass extends MyClass {
@Override
public String method(String param1) {
return "method with string parameter | " + super.method(param1);
}
}
In this case, we override method(String param1)
with same method name and parameters of its parent.
public class App {
public static void main(String[] args) {
MyClass myClass = new MyChildClass();
String result1 = myClass.method();
String result2 = myClass.method("parameterVal");
String result3 = myClass.method(1);
System.out.println("result1: " + result1); // result1: method with no parameter
System.out.println("result2: " + result2); // result2: method with string parameter | method with one parameter
System.out.println("result3: " + result3); // result3: method with an int parameter
}
}
Only result2
will use method of MyChildClass
because we have overrided it, other methods will use method of parent class.
In contrast with overloading, reference type will determine which method will be used at runtime for overriding.
Annotation @Override
As java specification:
Indicates that a method declaration is intended to override a method declaration in a supertype. If a method is annotated with this annotation type compilers are required to generate an error message unless at least one of the following conditions hold: - The method does override or implement a method declared in a supertype. - The method has a signature that is override-equivalent to that of any public method declared in Object.