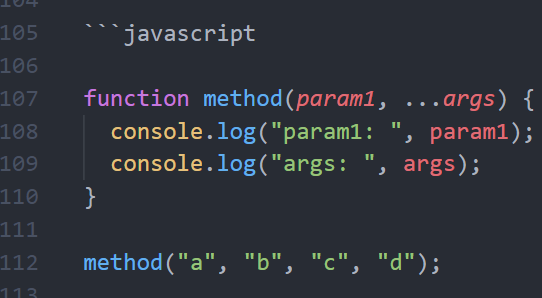
Optional parameters is the way for using method without required full all parameters in definition of method. It will be useful when using method in different contexts.
Java
In Java, we have two kinds of optional parameter of method:
- Overload methods
- Rest parameters
Java: Overload methods
In three methods below, we have different parameters for each method with the same method name:
import java.util.*;
import java.lang.*;
public class App {
private void method(){
System.out.println("method without parameters");
}
private void method(String param1){
System.out.println("method one parameter: " + param1);
}
private void method(String param1, String param2){
System.out.println("method two parameters: " + param1 + ", " + param2);
}
public static void main(String[] args){
App app = new App();
app.method();
app.method("a");
app.method("a", "b");
}
}
/**
Output:
method without parameters
method one parameter: a
method two parameters: a, b
**/
Java: Rest parameters
In below method, we use syntax String... params
to use optional parameters for method:
import java.util.*;
import java.lang.*;
public class App {
private void method(String... args) {
System.out.println("method with optional paramters, size: " + args.length + ", args: ");
for (String argv : args) {
System.out.println(argv);
}
}
public static void main(String[] args){
App app = new App();
app.method();
app.method("a");
app.method("a", "b");
}
}
/**
Output:
method with optional paramters, size: 0, args:
method with optional paramters, size: 1, args:
a
method with optional paramters, size: 2, args:
a
b
**/
Javascript
In javascript, there are many ways to use optional parameter: rest parameter, optional parameter, object parameter
Javascript: Rest parameter
Rest parameter in javascript is similar with rest parameter in java:
function method(param1, ...args) {
console.log("param1: ", param1);
console.log("args: ", args);
}
method("a", "b", "c", "d");
/**
Output:
param1: a
args: (3) ["b", "c", "d"]
*/
rest parameter will collect all remain paramter to this parameter (args
in this case)
Javascript: Optional parameter
In javascript, method has a dynamic execution, it does not concern to parameters when invoking, it will consider definition of method to get value of parameters,
function method(param1, param2 = "money", param3, param4){
console.log("param1: ", param1);
console.log("param2: ", param2);
console.log("param3: ", param3);
console.log("param4: ", param4);
}
method();
method("first");
method("first", "second");
method("a", "b", "c");
/**
Output:
param1: undefined
param2: money
param3: undefined
param4: undefined
param1: first
param2: money
param3: undefined
param4: undefined
param1: first
param2: second
param3: undefined
param4: undefined
param1: a
param2: b
param3: c
param4: undefined
*/
Javascript: Object parameter
Object parameter is a kind of Destructuring in javascript, mean that destructuring parameter in method definition. So we can use parameter name simply, and make sense.
function method({param1, param2 = "park", param3}) {
console.log("param1: ", param1);
console.log("param2: ", param2);
console.log("param3: ", param3);
}
method({}); // must use empty object like that to void undefined when read parameters of destructuring
method({param1: "first"});
method({param1: "first", param3: "third"});
method({param1: "first", param3: "third", param2: "second"});
/*
Output:
param1: undefined
param2: park
param3: undefined
param1: first
param2: park
param3: undefined
param1: first
param2: park
param3: third
param1: first
param2: second
param3: third
*/
C#
C# also supports many ways to use optional parameters:
- Rest parameter
- Overload method
- Named parameter
C#: Rest parameter:
Similar to Javascript or Java when using rest parameter:
using System;
class Program
{
static void method(params String[] args) {
Console.WriteLine("Method --->");
foreach(String argv in args){
Console.WriteLine("argv: " + argv);
}
}
static void Main() {
method();
method("a");
method("a", "b");
}
}
/*
Output:
Method --->
Method --->
argv: a
Method --->
argv: a
argv: b
*/
C#: Overload paramter
Similar to Java:
using System;
class Program
{
static void method() {
Console.WriteLine("Method ---> 0 parameter");
}
static void method(String param1) {
Console.WriteLine("Method ---> 1 parameter: " + param1);
}
static void method(String param1, String param2, String param3 = "you") {
Console.WriteLine("Method ---> optional parameters: " + param1 + ", " + param2 + ", " + param3);
}
static void Main() {
method();
method("a");
method("a", "b");
}
}
/**
Output:
Method ---> 0 parameter
Method ---> 1 parameter: a
Method ---> optional parameters: a, b, you
*/
C#: Named parameter
C# has a way to define method very interesting and similar to object parameter of javascript, but without object input, and required enough constraint parameter:
using System;
class Program
{
static void method(String param1, String param2, String param3 = "you") {
Console.WriteLine("Method ---> optional parameters: " + param1 + ", " + param2 + ", " + param3);
}
static void Main() {
method("first", "9");
method("flower", param2: "a");
method("a", "b", param3: "b");
}
}
/**
Output:
Method ---> optional parameters: first, 9, you
Method ---> optional parameters: flower, a, you
Method ---> optional parameters: a, b, b
**/