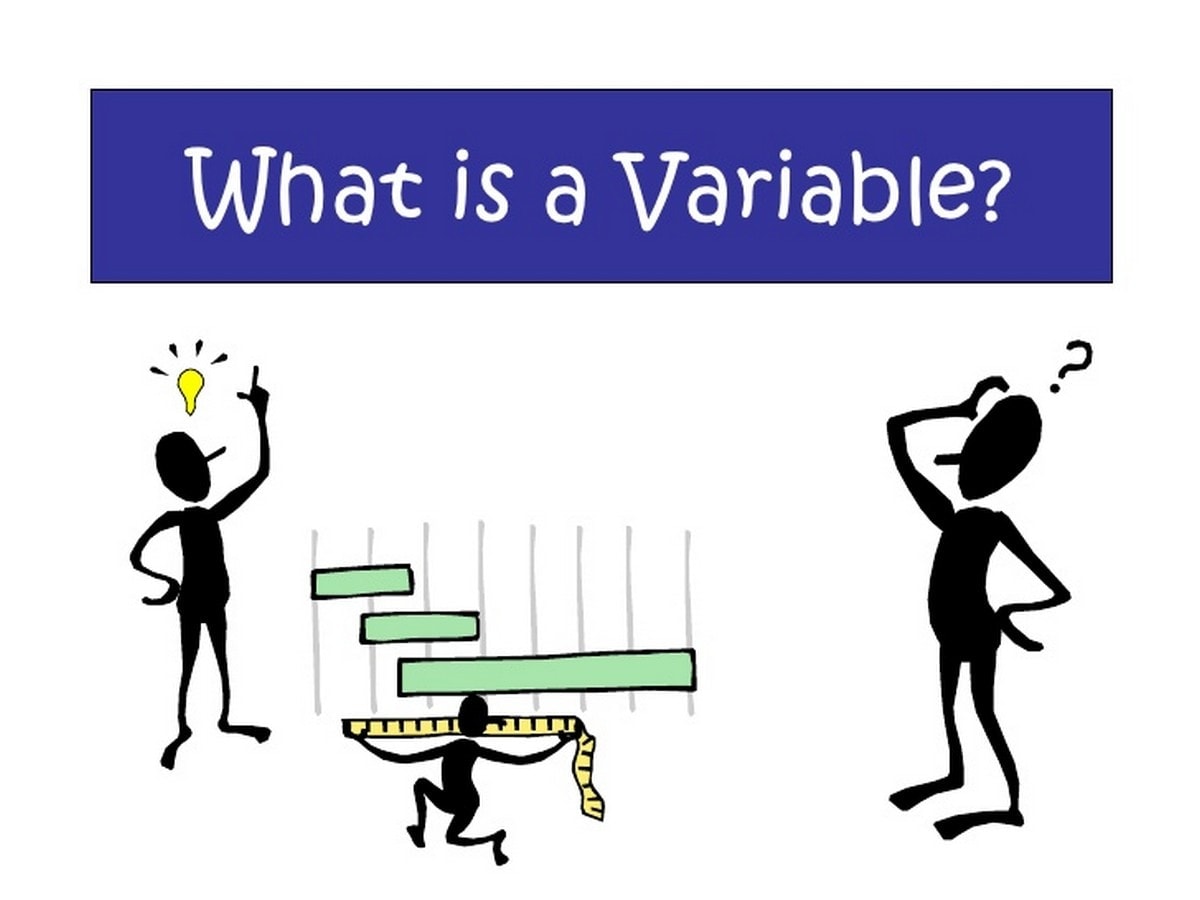
Variable declaration ways:
Along with the developer of javascript each year, the variable declaration has also made new improvements.
var
: the traditional variable declaration.let
: the variable declaration from ES5.const
: the variable declaration from ES5, immutable value.- No keyword declaration.
Difference between var
and let
:
Declaring variables with var
is a fairly arbitrary way of declaring
Able to re-declaring a declared variable:
var val = 9;
var val = 90;
console.log(val); // 90
Scope: where to use a variable
var
’s scope is function, meaning it will exist in function which it belongs to.
function fn() {
var x = 9;
{
console.log(x); // 9
}
console.log(x); // 9
}
fn();
console.log(x); // ReferenceError
let
’s scope is enclosed between{
and}
function fn() {
let x = 9;
{
console.log(x); // 9
let y = 10;
console.log(y); // 10
}
console.log(x); // 9
console.log(y); // ReferenceError
}
fn();
console.log(x); // ReferenceError
console.log(y); // ReferenceError
And because of this, in a loop (e.g. for
, while
) will easily lead to sometimes unpredictable contexts of var
.
Running through a loop can still reuse the variable in the loop:
for (var index = 0; index < 10; ++index) {
var val = index * 2;
console.log(val); // 0, 2, 4, 6, 8, 10, 12, 14, 16, 19
}
console.log(index); // 10
console.log(val); // 18
How to run the callback function:
for (var index = 0; index < 10; ++index) {
var val = index * 2;
setTimeout(function () {
console.log(val);
}, 0);
}
// Output: 18, 18, 18, 18, 18, 18, 18, 18, 18, 18
The function in setTimeout
is a callback function, meaning it will run after running the for loop, whether its timeout is zero.
And now, when the for
loop ends, the value of index
is 10, the value of variable val
will be 18, 10 functions in setTimeout
are invoked with the value of val = 18
, and lead to such result. Other way, when functions are run, it uses the reference of variable val
, but not the value in the for
loop.
We could change var
to let
to get the correct result. Because if it is declared with let
, the scope of variable val
will enclosed in the for loop, will not be changed value when looping, every time run through the next loop, the val
variable will be newly created (or have a new reference).
Or editable such like this:
for (var index = 0; index < 10; ++index) {
var val = index * 2;
setTimeout(function (val2) {
console.log(val2);
}, 0, val);
}
// Output: 0, 2, 4, 6, 8, 10, 12, 14, 16, 18
The value of variable val2
is been scope in its own function, so not affected from outside.
Hoisting
Variable of var
can use without delaring, but let
will raise error ReferenceError
:
function fn1(){
console.log(val); // undefined
var val = 10;
console.log(val); // 10
}
function fn2(){
console.log(val); // ReferenceError
let val = 10;
console.log(val); // 10
}
fn1();
fn2();
In the top level, var
wil created a global attribute, and let
won’t created.
In the top level in here meaning the variables declaring with var
and/or let
are not in any functions or blocks.
var name = "John";
let age = 24;
console.log(window.name); // John
console.log(window.age); // undefined
Declaring without keyword
When declare without keyword, it will be assign to the global attribute of window.
x = 9;
console.log(x); // 9
console.log(window.x); // 9
Declaring variable without keyword is like to assign window["x"] = 9
But not similar with var
, if we use x without declaring will raise error.
console.log(x); // ReferenceError
x = 9;
const
- The only difference between
let
andconst
is that the variable declared withconst
will not be able to be reassigned. - Nếu ta khai báo biến với
const
mà không cung cấp giá trị ngay lúc khai báo thì cũng sẽ gây lỗiSyntaxError
- If we declare a variable with
const
without giving it a value at the time of declaration, it will also causeSyntaxError
- The immutable value of
const
variable is only relative, i.e. you just cannot change it with the direct assignment=
, and if it is an object, you can change its properties, like this:
const info = {
name: "Luis",
age : 9
};
info.age += 1;
console.log(info.age); // 10
Destructure
We can also declare variable along the destructure process of the right side, for example:
let [a, b, ...rest] = [5, 8, 1, 4];
console.log(a); // 5
console.log(b); // 8
console.log(rest); // [1, 4]
let {name, age: mAge, ...rest2} = {
name: "Naln",
age: 10,
gender: "F",
address: "HCM City"
}
console.log(name); // Naln
console.log(mAge); // 10
console.log(rest2); // {gender: "F", address: "HCM city"}
And that is something I want to talk about variable declarations in javascript!