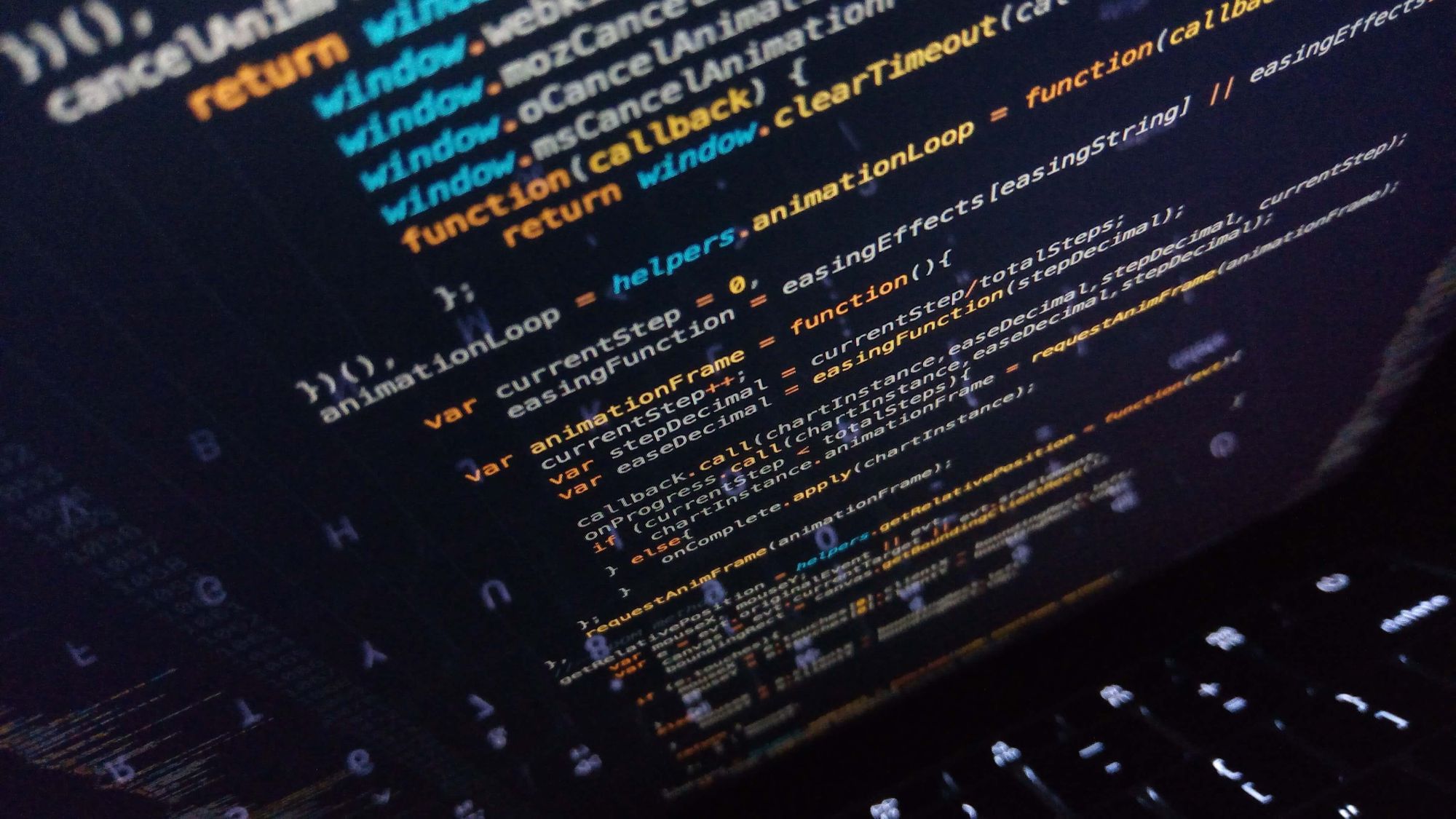
Declare a function
Declare a function to use in this post
function info(firstname, lastname, age){
console.log(firstname);
console.log(lastname);
console.log(age);
}
Popular way
As you know, we can invoke a function with syntax function_name(arg1, arg2)
. In our example, it will be:
info("Rick", "Thomas", 30);
// Output:
// Rick
// Thomas
// 30
What happen if we leave empty the final argument?
Its value will be
undefined
info("Rick", "Thomas");
// Output:
// Rick
// Thomas
// undefined
So, could we ignore the first agrument?
Yes, possible, but we will use another way to invoke,
apply
, you can see it in below.
In this way, to ignore first argument, we have to put it explicit undefined
value:
info(undefined, "Thomas", 30);
// Output:
// undefined
// Thomas
// 30
And not simple like that,
Even if you do not put any parameters in declare function, you can get it via variable arguments
:
function info(){
console.log(arguments);
}
info("Rick", "Thomas", 30);
// Output: ["Rick", "Thomas", 30]
// interesting? yeah
So, what if we also declare parameters for this function?
function info(firstname, lastname, age){
console.log(firstname);
console.log(lastname);
console.log(age);
console.log(arguments);
}
info("Rick", "Thomas", 30);
// Output:
// Rick
// Thomas
// 30
// ["Rick", "Thomas", 30]
That is, arguments
is always exists and represent for all arguments you put into function. Other way, arguments
is an attribute of Function
class.
Invoke a function via call
call
function is a method of object function
, or a method of Function
class: info.call(thisArgs, ...argsArr);
info.call(null, "Rick", "Thomas", 30);
// Output:
// Rick
// Thomas
// 30
You see, we have this
argument in this way, how to use it?
To use, in function, we just use
this
to do something like this:
function grow(name, luckyMoney){
console.log(`Give ${name} ${luckyMoney}$`);
this.money += luckyMoney;
}
let boy = {
name: "Lux",
money: 12
}
grow.call(boy, boy.name, 10); // Give Lux 10 $
console.log(boy);
// Output: {name: "Lux", money: 22}
Invoke a function via apply
Similar with call
, apply
is also a method of Function
class, but have different way to invoke:
info.apply(thisArgs, [ardsArr])
info.call(null, ["Rick", "Thomas", 30]);
// Output:
// Rick
// Thomas
// 30
As above mentioned, we invoke function and ignore the first parameter will look like this:
info.call(null, [,"Thomas", 30]);
// Output:
// underfine
// Thomas
// 30
Bonus,
Another method, not invoke function, just transfer it, that’s bind
info.bind(thisArgs, ...args)
Example we declare add
function like that:
function add(a, b){
return a + b;
}
we can bind it to a variable look like:
const add2 = add.bind()
add2(4, 5); // 9
What is different with assign it?
Yeah, it is almost the same, except that you can put something when
bind
it, to make it more powerful.
Some other interesting examples about bind
:
Ex1:
function add(a, b){
return a + b;
}
const add2 = add.bind(null, 2);
const add3 = add.bind(null, 3);
add2(5); // 7
add3(5); // 8
Ex2:
function getFullname(){
return `${this.firstname} ${this.lastname}`;
}
const boy = {
firstname: "John",
lastname: "Thomas"
};
const boyFullname = getFullname.bind(boy);
boyFullname(); // John Thomas
boy.firstname = "Meli";
boyFullname(); // Meli Thomas
Ex3:
function add(a, b){
return a + a;
}
const addAssign = addFunc;
const addBind = addFunc.bind();
addAssign(7,8); // 14
addBind(7,8); // 14
function add(a, b){
return a + b;
}
addAssign(7,8); // 14
addBind(7,8); // 14
// That's, they are not changed when redefining `add` function
So, there are many ways to invoke a function. It depends on the context and used accordingly. :)